This tutorial will walk you through the steps to create a 3D pie chart with two data series. Pie data is read through data binding.
1. Create the pie chart
You can create the chart in the XAML editor by dragging the icon for the 3D pie chart and dropping it at your work area. Alternatively, you can create the chart through code:
C#
Copy Code
|
---|
PieChart3D pChart = new PieChart3D(); Grid.SetRow(pChart, 0); Grid.SetColumn(pChart, 0); myGrid.Children.Add(pChart); |
VB.NET
Copy Code
|
---|
Dim pChart As PieChart3D = New PieChart3D Grid.SetRow(pChart, 0) Grid.SetColumn(pChart, 0) myGrid.Children.Add(pChart) |
2. Create the PieSeries
Each chart has initially a default series - we clear it and create our two pie series from scratch:
C#
Copy Code
|
---|
pieChart3D1.Series.Clear();
PieSeries series1 = new PieSeries(); pieChart3D1.Series.Add(series1);
PieSeries series2 = new PieSeries(); pieChart3D1.Series.Add(series2); |
VB.NET
Copy Code
|
---|
pieChart3D1.Series.Clear
Dim series1 As PieSeries = New PieSeries pieChart3D1.Series.Add(series1)
Dim series2 As PieSeries = New PieSeries pieChart3D1.Series.Add(series2) |
The data for the pie chart is taken from an ObservableCollection called sales. It has two fields - Profit and Turnover that will provide data for the pie series:
C#
Copy Code
|
---|
pieChart3D1.DataSource = "sales";
series1.DataPath = "Profit"; series2.DataPath = "Turnover"; |
VB.NET
Copy Code
|
---|
pieChart3D1.DataSource = "sales"
series1.DataPath = "Profit" series2.DataPath = "Turnover" |
3. Set the labels
We want to show the percent value for each slice inside it. For this we use the InnerLabelType property. We adjust the location of the labels with InnerLabelLocation. The font is bold.
C#
Copy Code
|
---|
series1.InnerLabelLocation = 0.7; series1.InnerLabelType = LabelType.Percents; series1.LabelFontWeight = FontWeights.Bold; |
VB.NET
Copy Code
|
---|
series1.InnerLabelLocation = 0.7 series1.InnerLabelType = LabelType.Percents series1.LabelFontWeight = FontWeights.Bold |
4. Customizing the appearance
We increase the thickness of the doughnut series with DoughnutThickness and create a set of brushes for the slices:
C#
Copy Code
|
---|
pieChart3D1.DoughnutThickness = 2;
List<Brush> brushes = new List<Brush>(); brushes.Add(Brushes.LightBlue); brushes.Add(Brushes.LightGreen); brushes.Add(Brushes.LightPink); brushes.Add(Brushes.LightYellow); brushes.Add(Brushes.LightSalmon); brushes.Add(Brushes.LightCyan);
series1.Fills = brushes; series2.Fills = brushes; |
VB.NET
Copy Code
|
---|
pieChart3D1.DoughnutThickness = 2
Dim brushes As List(Of Brush) = New List(Of Brush) brushes.Add(Brushes.LightBlue) brushes.Add(Brushes.LightGreen) brushes.Add(Brushes.LightPink) brushes.Add(Brushes.LightYellow) brushes.Add(Brushes.LightSalmon) brushes.Add(Brushes.LightCyan)
series1.Fills = brushes series2.Fills = brushes |
Here is the final chart:
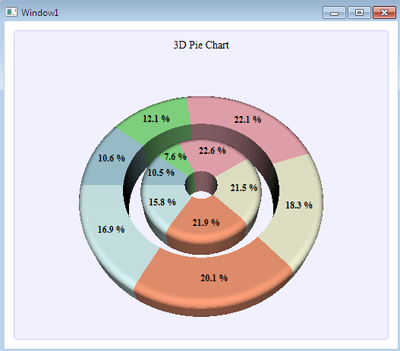