This tutorial shows how to load hierarchical data recursively from XML and create diagram items corresponding to the hierarchy.
1. Right-click the project in Solution Explorer and choose Add -> New Item from the context menu. Create a new XML file called SampleTree.xml and add to it content in the following form:
XML
Copy Code
|
---|
<?xml version="1.0" encoding="utf-8" ?> <Project> <Activity Name="Activity 1"> <Activity Name="sub-activity 1"> <Activity Name="sub-activity 1-1" /> <Activity Name="sub-activity 1-2" /> </Activity> <Activity Name="sub-activity 2"> <Activity Name="sub-activity 2-1" /> <Activity Name="sub-activity 2-2" /> <Activity Name="sub-activity 2-3" /> </Activity> <Activity Name="sub-activity 3"> <Activity Name="sub-activity 3-1" /> <Activity Name="sub-activity 3-2" /> </Activity> </Activity> <Activity Name="Activity 2"> <Activity Name="sub-activity 1"> <Activity Name="sub-activity 1-1" /> <Activity Name="sub-activity 1-2" /> <Activity Name="sub-activity 1-3" /> </Activity> <Activity Name="sub-activity 2"> <Activity Name="sub-activity 2-1" /> <Activity Name="sub-activity 2-2" /> </Activity> </Activity> <Activity Name="Activity 3"> <Activity Name="sub-activity 1"> <Activity Name="sub-activity 1-1" /> <Activity Name="sub-activity 1-2" /> <Activity Name="sub-activity 1-3" /> <Activity Name="sub-activity 1-4" /> </Activity> <Activity Name="sub-activity 2" /> <Activity Name="sub-activity 3"> <Activity Name="sub-activity 3-1" /> <Activity Name="sub-activity 3-2" /> </Activity> </Activity> </Project> |
2. Set the file's "Build Action" property to "Content" and "Copy to Output Directory" to "Copy if newer".
3. Add a Loaded event handler to the WPF window:
Xaml
Copy Code
|
---|
<Window ... Loaded="OnWindowLoaded" ...> |
C#
Copy Code
|
---|
private void OnWindowLoaded(object sender, RoutedEventArgs e) { } |
4. Add the following member to the window class to hold the default node size.
C#
Copy Code
|
---|
Rect nodeBounds = new Rect(0, 0, 100, 22); |
5. Create the tree root node, and call the CreateChildren method, which builds the tree recursively.
C#
Copy Code
|
---|
var root = diagram.Factory.CreateShapeNode(nodeBounds); root.Text = "Project"; var document = XDocument.Load("SampleTree.xml"); CreateChildren(root, document.Element("Project")); |
6. Define CreateChildren as follows. It takes as parameters the parent DiagramNode and parent XML element, iterates the child XML elements to build the next level of the hierarchy, and creates corresponding diagram items by calling CreateShapeNode and CreateDiagramLink.
C#
Copy Code
|
---|
private void CreateChildren(DiagramNode parentDiagNode, XElement parentXmlElement) { foreach (var element in parentXmlElement.Elements("Activity")) { var node = diagram.Factory.CreateShapeNode(nodeBounds); node.Text = element.Attribute("Name").Value; diagram.Factory.CreateDiagramLink(parentDiagNode, node); CreateChildren(node, element); } } |
7. Use TreeLayout to arrange the diagram. Add the following code to the end of the Loaded handler.
C#
Copy Code
|
---|
var layout = new TreeLayout(); layout.Type = TreeLayoutType.Cascading; layout.Direction = TreeLayoutDirections.LeftToRight; layout.LinkStyle = TreeLayoutLinkType.Cascading2; layout.NodeDistance = 5; layout.LevelDistance = -25; // let horizontal positions overlap layout.Arrange(diagram); |
8. Build and run the project. If everything is fine, you should see this representation of the hierarchy:
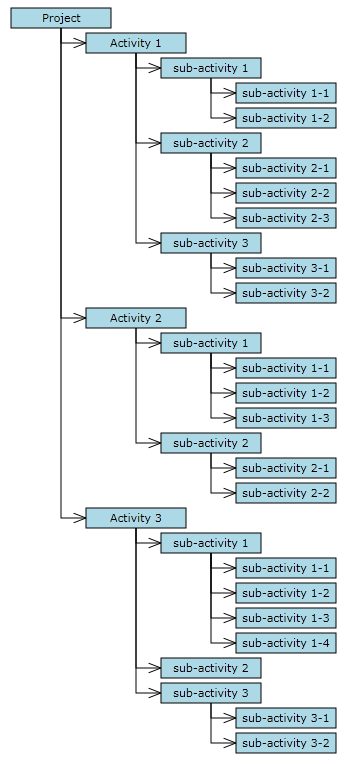