With Charting for WPF you can:
- Create custom themes based on the appearance of an existing chart;
- Save a custom theme as an XML file;
- Load a custom theme from its XML file;
- Use a number of predefined themes.
1. Create a custom theme based on the appearance of an existing chart
Style your chart as you want. In our sample we will use a BarChart. We set a background, font settings for the title, brushes for the grid and customize the outline of the chart:
C#
Copy Code
|
---|
BarChart modelChart = new BarChart() { Background = Brushes.Beige, BorderThickness = new Thickness(5), CornerRadius = new CornerRadius(5), TitleFontSize = 18, TitleFontWeight = FontWeights.Bold, TitleFontFamily = new FontFamily("Tahoma"), GridFills = new List<Brush>() { Brushes.LightGray, Brushes.Gray }, GridStrokes = new List<Brush>() { Brushes.DarkGray }, PlotAreaStroke = Brushes.DarkGray }; |
VB.NET
Copy Code
|
---|
Dim modelChart As New BarChart() With { _ .Background = Brushes.Beige, _ .BorderThickness = New Thickness(5), _ .CornerRadius = New CornerRadius(5), _ .TitleFontSize = 18, _ .TitleFontWeight = FontWeights.Bold, _ .TitleFontFamily = New FontFamily("Tahoma"), _ .GridFills = New List(Of Brush)() From { _ Brushes.LightGray, _ Brushes.Gray _ }, _ .GridStrokes = New List(Of Brush)() From { _ Brushes.DarkGray _ }, _ .PlotAreaStroke = Brushes.DarkGray _ } |
Customize the appearance of the BarSeries. In the sample we add 4 brushes and strokes - one for each bar, because we are showing four bars.
C#
Copy Code
|
---|
//create a new series and style it. BarSeries series = new BarSeries() { StrokeThickness = 2, };
series.Fills.Add(Brushes.Red); series.Fills.Add(Brushes.Green); series.Fills.Add(Brushes.Blue); series.Fills.Add(Brushes.Violet); series.Strokes.Add(Brushes.DarkRed); series.Strokes.Add(Brushes.DarkGreen); series.Strokes.Add(Brushes.DarkBlue); series.Strokes.Add(Brushes.DarkViolet);
//add the series to the series collection modelChart.Series.Add(series); |
VB.NET
Copy Code
|
---|
'create a new series and style it. Dim series As New BarSeries() With { _ .StrokeThickness = 2 _ }
series.Fills.Add(Brushes.Red) series.Fills.Add(Brushes.Green) series.Fills.Add(Brushes.Blue) series.Fills.Add(Brushes.Violet) series.Strokes.Add(Brushes.DarkRed) series.Strokes.Add(Brushes.DarkGreen) series.Strokes.Add(Brushes.DarkBlue) series.Strokes.Add(Brushes.DarkViolet)
'add the series to the series collection modelChart.Series.Add(series) |
We finally create a new ChartTheme based on the model and we apply this theme to the chart we already have - barChart1.
C#
Copy Code
|
---|
//create the theme ChartTheme theme = new ChartTheme(modelChart);
//apply the theme theme.Apply(barChart); |
VB.NET
Copy Code
|
---|
'create the theme Dim theme As New ChartTheme(modelChart)
'apply the theme theme.Apply(barChart) |
2. Save a custom theme as a XML file
You do this by calling the ChartTheme.SaveToXml method and providing the name of the xml file.
C#
Copy Code
|
---|
theme.SaveToXml("sampleTheme.xml"); |
VB.NET
Copy Code
|
---|
theme.SaveToXml("sampleTheme.xml") |
3. Load a theme from a XML file
You load a theme with the ChartTheme.LoadFromXml method. The method takes one argument, which is the name of the XML file.
C#
Copy Code
|
---|
barChart.LoadTheme("C:\\Users\\UserName\\Downloads\\Blue.xml"); |
VB.NET
Copy Code
|
---|
barChart.LoadTheme("C:\Users\UserName\\Downloads\Blue.xml") |
4. Predefined themes
Charting for WPF offers a set of predefined themes, which are found in the setup directory. You can load them the way you would load any XML ChartTheme - see point 3.
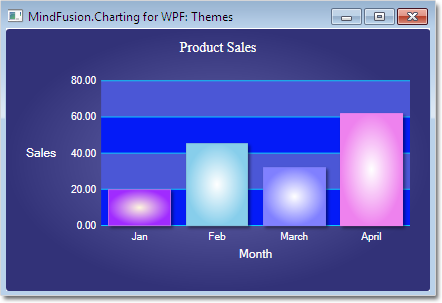
The predefined Blue theme applied on a bar chart.