The list below describes recent changes and additions to NetDiagram:
New in version 7
ES6 classes and properties
(client side / JavaScript)
Client side scripts have been refactored to use ES6 classes, properties and native collections. ArrayList, Dictionary and Set classes from MindFusion.Collections namespace have been removed, and replaced by respective JavaScript native Array, Map and Set. Get/set functions have been replaced by getter/setter properties, which should help using the diagram API with binding expressions of various JS frameworks. You could enable propFunctions in CompatConfig in order to continue using legacy get/set functions during migration.
Diagram view
(client side / JavaScript)
The Diagram JavaScript class has been refactored into separate Diagram and DiagramView classes, matching respective server-side .NET classes that the library already provided in older versions. What remains of Diagram is now just a model class that defines diagram structure and implements serialization. The new DiagramView class deals with rendering and user interaction. You can show the same diagram in several views, each one rendering at different scroll positions and zoom levels. Note that you can no longer call Diagram.find(id) on client-side, but instead call DiagramView.find(id).diagram to access the Diagram object.
TreeView nodes
The TreeViewNode class represents nodes that can display hierarchical data. The root items displayed in the node can be accessed through the rootItems property. Items can be added and removed individually by using the addItem and removeItem methods, or in bulk by calling the fromObject method, which loads the tree view items from an array of objects.
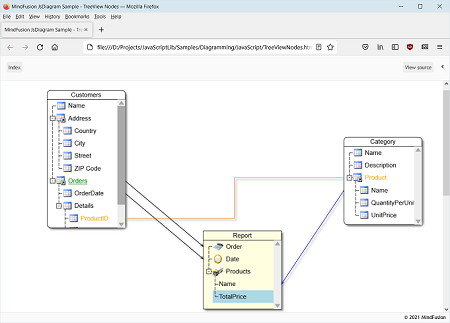
Print pagination
(client side / JavaScript)
The printPreview and print methods of DiagramView let you export the diagram as a list of smaller images in HTML page. Supported options include printArea (defaults to diagram's content bounds) and pageSize (defaults to DiagramView's viewport). Note that print methods use HTMLCanvasElement.toDataURL() internally, so any limitations it has will apply (such as canvas size and CORS).
Client-side layout
- The RadialTreeLayout class arranges tree levels in concentric circles around the root. The levelDistance property specifies the distance between adjacent circles. The direction property specifies placement of first child node. Set stretchFactor to a value larger than 1 in order to stretch level circles into ellipses, providing more space for the layout when using wider nodes.
- TopologicalLayout can now be applied on client-side.
- OrthogonalLayout can now be applied on client-side.
Multiple labels per node
The NodeLabel class allows multiple captions to be displayed for a single DiagramNode of any type. Node labels provide a set of properties allowing full customization of their display and positioning. Label position is defined by specifying a pin point and offset from it, set through SetCornerPosition, SetEdgePosition, SetCenterPosition methods. In addition, the HorizontalAlign and VerticalAlign properties of NodeLabel specify on which side of pin point to draw the label's caption.
For example, following code adds extra labels to top-left and bottom-right corners of a ShapeNode:
C#
Copy Code
|
---|
var node = diagram.Factory.CreateShapeNode(10, 50, 40, 30); node.Text = "text"; // centered main text
var lb1 = node.AddLabel("label 1"); lb1.SetCornerPosition(0, 0, 0); lb1.HorizontalAlign = StringAlignment.Near; lb1.VerticalAlign = StringAlignment.Near; lb1.Brush = new SolidBrush(Color.Red); lb1.TextBrush = new SolidBrush(Color.White);
var lb2 = node.AddLabel("label 2"); lb2.SetCornerPosition(2, 0, 0); lb2.HorizontalAlign = StringAlignment.Far; lb2.VerticalAlign = StringAlignment.Far; lb2.Brush = new SolidBrush(Color.Yellow); lb2.TextBrush = new SolidBrush(Color.Red); |
Multi-touch support
- If MultiTouchZoom property is enabled (default), the view can be zoomed or panned using two-touch pinch / flick gestures.
- If MultiTouchModify property is enabled (default), diagram nodes can be moved, scaled and rotated using two-touch pinch / flick gestures.
- If MultiTouchZoom property is disabled, each touch draws diagram items corresponding to current behavior.
- If MultiTouchModify property is disabled, each touch started from a node draws a diagram link.
- Latter modes can be used for collaborative whiteboarding / classroom scenarios.
- The MultiTouchDraw property of DiagramView lets you prevent drawing multiple items simultaneously, while keeping other multitouch gestures enabled.
- The HandleTouchHitDistance property of DiagramView makes it easier to grab adjustment handles on mobile devices, without increasing the AdjustmentHandlesSize value.
Mouse input improvements
- MouseWheelAction property of DiagramView lets you choose between Scroll (default) and Zoom as the default behavior of the control in response of a wheel event.
- The MoveNodes behavior allows grabbing nodes to drag them without using adjustment handles.
- The control now captures mouse events and completes drag / drawing operations even if mouse is released outside the diagram view.
New events
Miscellaneous
- Client side createDiagramLink method now accepts two, three or four arguments, letting you specify row index for origin or destination TableNode, or TreeViewItem for origin or destination TreeViewNode.
- Clipboard methods now use modern Navigator.clipboard API when their systemClipboard argument is enabled.
- Rotation of FreeFormNode instances.
- ImageAlign supports new FitLeft, FitTop, FitRight and FitBottom alignment styles, which resize image to fit node's boundaries and align it to respective border.
- Fix for resizeToFitText not calculating correct size when cells have text padding applied.
- The EnumAllPaths method of PathFinder yields each path immediately when found.
- strokeDashArray and strokeDashOffset properties let you set custom dash patterns when strokeDashStyle is set to Custom.
- Styled text now supports <size=N> element letting you set font size.
- enableStyledText property added to ItemLabel lets you format labels using HTML-like format flags.
- enableWrap and maxWidth properties of ItemLabel let you wrap label's text.
- The ArrowHeads class exposes arrowhead shapes as static methods returning respective predefined shape.
- Fixed linkTextStyle exception.
- Fix for NodeListView not working correctly when hosted nodes contain NodeLabel objects.
- New PatternRouter constructor accepts string definitions of link routes.
- EnableParallelism and MaxDegreeOfParallelism layout properties now available in CoreCLR assemblies (.NET Core 3 and 5+ versions).
- New Export overloads of exporter classes allow writing to System.IO.Stream.
- The MoveLabels behavior lets user move link and node labels interactively.
- HorizontalOffset and VerticalOffset now work for link labels positioned using SetLinkLengthPosition method.
- ExcelExporter exports pen width of links.
- ExcelExporter sets more precise positions of link end points.
- LinkEventArgs now includes origin and destination properties that report respective candidate nodes to LinkCreating and LinkModifying client-side event handlers.
- SvgNode.ContentAlign property specifies alignment of SVG drawing inside the node.
- DiagramLink.lineAlignment property sets the vertical position of link's text.
- Horizontal scroll gestures should now work on Macbook trackpad when using virtual scroll mode.
JavaScript API changes
- getProperty/setProperty function pairs from client-side API have been replaced by ES6 getters/setters. Set CompatConfig.propFunctions to true in order to continue using legacy get/set functions in your code.
- Diagram.find method has been removed. Call DiagramView.find instead, and get the view's diagram property.
- Client-side scroll and zoom properties moved from Diagram to DiagramView: autoScroll, scrollZoneSize, autoScrollAmount, scrollX, scrollY, viewport, virtualScroll, zoomFactor.
- Client-side input related properties moved from Diagram to DiagramView: allowInplaceEdit, delKeyAction, behavior, tooltipDelay, modificationStart, modifierKeyActions, leftButtonActions, middleButtonActions, rightButtonActions.
- Client-side magnifier properties moved from Diagram to DiagramView: magnifierEnabled, magnifierFactor, magnifierWidth, magnifierHeight, magnifierFrameThickness, magnifierShading, magnifierShape,magnifierFrameColor, magnifierSecondaryFrameColor.
- Client-side methods moved from Diagram class to DiagramView class: create, find, record, stopRecording, replay, clearTooltip, beginEdit, copyToClipboard, pasteFromClipboard, cutToClipboard, scrollTo, zoomToRect, zoomToFit, setZoomFactorPivot.
- MindFusion.AbstractionLayer class has been removed.
- EventArgs and CancelEventArgs classes have been moved to the MindFusion.Controls namespace.
- ArrayList, Dictionary, ObservableCollection, Set classes have been removed from MindFusion.Collections namespace.
- Due to Navigator.clipboard API being asynchronous, pasted items are not available immediately after calling pasteFromClipboard with systemClipboard enabled. You will be able to access them only after nodePasted and linkPasted events are raised.
.NET API changes