We assume you have created a map as shown in the "Your First Map" tutorial. Now we are going to extend this map with different Decoration types that are present in the library - Bubble-s and Marker-s. We will see how they can be used to create location pins, explanation labels, images and header
text.
I. Images
Create a new DecorationLayer instance. The string parameter in the constructor provides the name for this layer. This name appears as a title for the layer in the layer controller of the map. We don't want the images to appear on the map by default so we set the visible property of the new layer to false. Finally we add it to the layers collection for the MapView:
JavaScript
Copy Code
|
---|
// add a decoration layer for marker images var markers = new m.DecorationLayer("Images"); markers.visible = false; view.layers.add(markers); |
Each image requires an instance of the
Marker class. The
imageSrc property points to the location of the image on your web server. The text provided through the text property is used for a tooltip:
The text of the image Marker appears as a tooltip when the user hovers over the image.If the
imageSrc property is not set the appearance of the
Marker should be defined in css, by setting its
cssClass property to your custom css class. If neither
imageSrc and
cssClass are set, a default css class will be added to the marker DOM element, namely "mfm-css-marker", which represents a pin shape.
We provide the location where this image shall appear on the map as a
LatLong value in the constructor. The
LatLong value speciies the geographical coordinates - latitude and longitude of the object:
JavaScript
Copy Code
|
---|
// create some markers with images var mark = new m.Marker(new m.LatLong(-22.951916, -43.210487)); mark.imageSrc = "./images/christ_redeemer.png"; mark.text = "Christ the Redeemer"; markers.decorations.add(mark); |
Then add the new Marker to the decorations property of the markers layer.
II. Pins
The pins are also instances of the Marker class. In order to create a pin we need to create just a new instance of the Marker class and set its location. We don't set an image or text. The pins will be on a new DecorationLayer and by default they are visible:
JavaScript
Copy Code
|
---|
// add a decoration layer for marker pins var pins = new m.DecorationLayer("Pins"); pins.visible = true; view.layers.add(pins); |
Then we use the forEach method of the
decorations collection and create a new simple
Marker e.g. pin for each image
Marker that is found in the markers list:
JavaScript
Copy Code
|
---|
// create some markers with default css styling var pin; markers.decorations.forEach( function (mark) { this.decorations.add(new m.Marker(mark.location)); }, pins); |
III. Info Bubbles
The Bubble class provides labels with text that can be on multiple lines or a single line. In our sample we will use both types of info text. We first create a new DecorationLayer that will hold info Bubble-s with detailed text about each place of interest:
JavaScript
Copy Code
|
---|
// add a decoration layer for info bubbles var bubbles = new m.DecorationLayer("Info bubbles"); bubbles.visible = false; view.layers.add(bubbles); |
The Bubble class again requires that the location be specified in the constructor. The text property accepts HTML formatting symbols. By default a Bubble renders text on multiple lines:
JavaScript
Copy Code
|
---|
// create some bubbles showing an HTML string var bubble = new m.Bubble(new m.LatLong(-22.951916, -43.210487)); bubble.text = "<p>Christ the Redeemer</p>The statue weighs 635 metric tons (625 long, 700 short tons), </br> and is located at the peak of the 700-metre (2,300 ft) Corcovado mountain </br> in the Tijuca Forest National Park overlooking the city of Rio de Janeiro. </br> A symbol of Christianity across the world, the statue has also become a cultural icon </br> of both Rio de Janeiro and Brazil, and is listed as one of the New 7 Wonders of the World."; bubbles.decorations.add(bubble); |
We add the new
Bubble to the
decorations property list of the bubbles layer, which is also not visible by default.
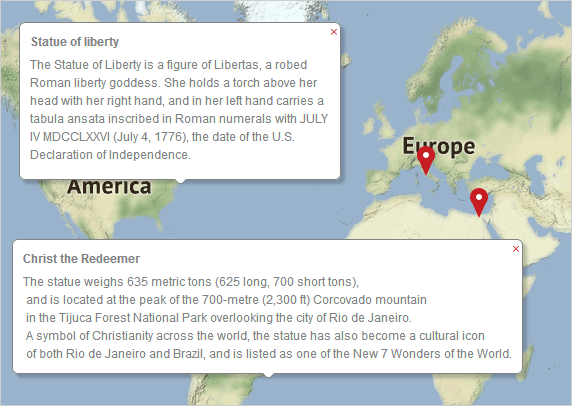
Multiline info Bubble-s are rendered at locations of interest. Users can close them by clicking on the 'x' button.
IV. Captions
The captions are also Bubble instances. We set the multiline property to false because we want the name of the object to appear on a single line. We use the short text that appears as a tooltip in the markers layer to appear as a caption:
JavaScript
Copy Code
|
---|
// add a decoration layer for caption bubbles var captions = new m.DecorationLayer("Captions"); captions.visible = false; view.layers.add(captions); // create some bubbles showing a one-line text var caption; markers.decorations.forEach( function (mark) { var caption = new m.Bubble(mark.location, mark.text); caption.multiline = false; this.decorations.add(caption); }, captions); |
We can specify a custom text formatting for the caption using the
cssClass property. We declare one in the <HEAD> section of the web page:
HTML
Copy Code
|
---|
.mfm-bubble.caption:before, .mfm-bubble.caption:after { border: none; }
|
Then we assign:
JavaScript
Copy Code
|
---|
caption.cssClass = "caption"; |
Let's shift the caption a little bit downwards because by default it will show over the image. We do this with the offset property, which requires a Point value:
JavaScript
Copy Code
|
---|
caption.offset = new d.Point(0, 30); |
That's how captions are rendered:
A map that shows pins with captions.