In this tutorial we will build the data-entry form shown below:
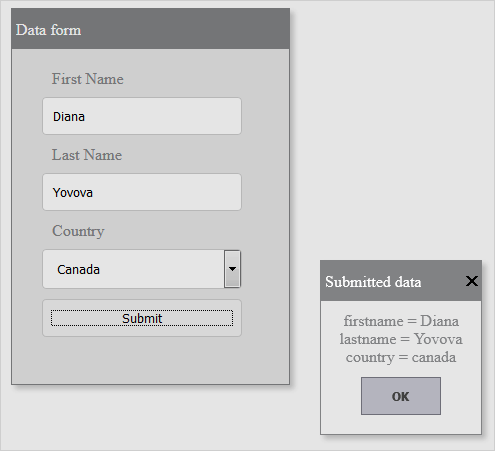
The form will be represented by an instance of the Window control.
I. References
In the HEAD section of the web page that will render the form we add following references:
HTML
Copy Code
|
---|
<script src="drawing.js" type="text/javascript"></script> <script src="controls.js" type="text/javascript"></script> <script src="common.js" type="text/javascript"></script> <script src="common-collections.js" type="text/javascript"></script> <script src="common-ui.js" type="text/javascript"></script> |
They contain shared code used throughout MindFusion.JavaScript Pack, and the last script implements the controls from MindFusion.Common.UI namespace. If using NPM, it is enough to add a reference to @mindfusion/common-ui package, and the other ones will get pulled as dependencies.
II. The Window Control
We create a mapping to the MindFusion.Common.UI namespace and then create a Window object:
JavaScript
Copy Code
|
---|
var ui = MindFusion.Common.UI; // Create a new Window. // If the element parameter of the Window constructor is not provided, the DOM element of the control will be created internally. var window1 = new ui.Window(); Note that each MindFusion.Common.UI Control instance requires an HTMLElement to be associated with. If no such element is provided a new <div> element will be created automatically. In such cases you need to add the Control to the DOM tree of the web page, between calling its draw and attach methods: // Draw the window and append it to the page DOM. document.body.children[1].appendChild(window1.draw()); // Prepare the window for user interaction. window1.attach(); |
III. Window Customization
The Window class exposes a rich set of properties for customizing the appearance of the control. We use some of them to adjust the looks and behavior of our Window instance:
JavaScript
Copy Code
|
---|
// Set the window title window1.title = "Data form"; // Set the window theme window1.theme = "standard"; // Set the window visibility to false to avoid flickering during content loading. window1.visible = false; // Disable interactions. window1.allowResize = false; window1.allowPin = false; window1.allowMaximize = false; window1.allowMinimize = false; window1.allowClose = false; // Set the window templateUrl. // The HTML of the specified page will be set as the innerHTML of a scrollable div inside the Window's content element. window1.templateUrl = "form.html"; |
The contents of the Window is loaded from a template. The template is a simple HTML page called form.html, which is placed in the same directory as the page with the data-entry form. The form page contains the HTML of a web form:
HTML
Copy Code
|
---|
<!DOCTYPE html> <html> <body> <form id="form"> <label for="fname">First Name</label> <input type="text" id="fname" name="firstname" placeholder="Your name.."> <label for="lname">Last Name</label> <input type="text" id="lname" name="lastname" placeholder="Your last name.."> <label for="country">Country</label> <select id="country" name="country"> <option value="australia">Australia</option> <option value="canada">Canada</option> <option value="usa">USA</option> </select> <input type="submit" id="submit" value="Submit"> </form> </body> </html> |
IV. Events and Event Handling
We handle the contentLoad event of the Window class class by attaching an event handler method:
JavaScript
Copy Code
|
---|
// Add event handlers. window1.contentLoad.addEventListener(contentLoad); |
The event handler receives two arguments - one is the sender, which is the Window that we created. The other is an event args object. In this case the
EventArgs object is of type
EmptyEventArgs and does not contain any informaton about the event.
JavaScript
Copy Code
|
---|
function contentLoad(sender, args) { sender.visible = true; sender.autoSize(); // Attach a handler to the form submit event. sender.element.querySelector("#form").addEventListener("submit", postData.bind(sender)); } |
When the contentLoad event is raised we make the form visible, adjust its size automatically and attach an event handler to the submit event of the HTML form.
When the user presses the submit button the event handler takes the user input and creates an InfoDialog that renders it:
JavaScript
Copy Code
|
---|
function postData(e) { // Prevent form submission. e.preventDefault(); // Get a reference to the form element and collect the data. var form = this.element.querySelector("form"); var data = ""; for (var i = 0; i < form.elements.length - 1; i++) { data += form.elements[i].name + " = " + form.elements[i].value + "\n"; } // Show an information box with the submitted data and dispose the window. ui.Dialogs.showInfoDialog("Submitted data", data, function () { this.dispose(); }.bind(this), document.body.children[1], "standard"); } |
The showInputDialog method disposes the dialog, sets the current window as the parent element and colors it with the standard theme.