This tutorial shows how to load hierarchical data recursively from a Json string and create diagram items corresponding to the hierarchy. The code is also available in Samples folder of JsDiagram distribution.
1. Create a text file called Tutorial2.txt and add the following Json string to it:
XML
Copy Code
|
---|
{ "project" : {"name": "project", "activities":[ {"name":"Activity 1", "activities":[ {"name":"sub-activity 1", "activities":[ {"name":"sub-activity 1-1"}, {"name":"sub-activity 1-2"}]}, {"name":"sub-activity 2", "activities":[ {"name":"sub-activity 2-1"}, {"name":"sub-activity 2-2"}, {"name":"sub-activity 2-3"}]}, {"name":"sub-activity 3", "activities":[ {"name":"sub-activity 3-1"}, {"name":"sub-activity 3-2"}]} ]}, {"name":"Activity 2", "activities":[ {"name":"sub-activity 1", "activities":[ {"name":"sub-activity 1-1"}, {"name":"sub-activity 1-2"}, {"name":"sub-activity 1-3"}]}, {"name":"sub-activity 2", "activities":[ {"name":"sub-activity 2-1"}, {"name":"sub-activity 2-2"}]} ]}, {"name":"Activity 3", "activities":[ {"name":"sub-activity 1", "activities":[ {"name":"sub-activity 1-1"}, {"name":"sub-activity 1-2"}, {"name":"sub-activity 1-3"}, {"name":"sub-activity 1-4"}]}, {"name":"sub-activity 2"}, {"name":"sub-activity 3", "activities":[ {"name":"sub-activity 3-1"}, {"name":"sub-activity 3-2"}, {"name":"sub-activity 3-3"}]} ]} ]} } |
2. Create empty Tutorial2.html and Tutorial2.js files, and add DIV and CANVAS elements to the HTML page.
HTML
Copy Code
|
---|
<!-- The DiagramView component is bound to the canvas element below --> <div style="width: 100%; height: 100%; overflow: auto;"> <canvas id="diagram" width="2100" height="2100"> This page requires a browser that supports HTML 5 Canvas element. </canvas> </div> |
3. Add script references to the MindFusion UMD scripts and Tutorial2 JavaScript files:
HTML
Copy Code
|
---|
<script src="umd/collections.js" type="text/javascript"></script> <script src="umd/drawing.js" type="text/javascript"></script> <script src="umd/controls.js" type="text/javascript"></script>
<script src="umd/animations.js" type="text/javascript"></script> <script src="umd/graphs.js" type="text/javascript"></script> <script src="umd/diagramming.js" type="text/javascript"></script>
<script src="Tutorial2.js" type="text/javascript"></script> |
4. In Tutorial2.js, assign shorter names to the DiagramView, Diagram, ShapeNode, DiagramLink, BorderedTreeLayout and Rect classes (if using diagram's ES6 or CommonJS modules, these would be import or require statements for respective classes and modules).
JavaScript
Copy Code
|
---|
var DiagramView = MindFusion.Diagramming.DiagramView; var Diagram = MindFusion.Diagramming.Diagram; var DiagramLink = MindFusion.Diagramming.DiagramLink; var ShapeNode = MindFusion.Diagramming.ShapeNode; var Rect = MindFusion.Drawing.Rect; var BorderedTreeLayout = MindFusion.Graphs.BorderedTreeLayout; |
5. Add a DOMContentLoaded handler that creates a DiagramView instance wrapping the HTML Canvas element:
JavaScript
Copy Code
|
---|
var diagram = null;
document.addEventListener("DOMContentLoaded", function () { // create a DiagramView component that wraps the "diagram" canvas var diagramView = DiagramView.create(document.getElementById("diagram")); diagram = diagramView.diagram; } |
6. Add the following members to the script to hold the default node size and color.
JavaScript
Copy Code
|
---|
var bounds = new Rect(0, 0, 30, 6); var brush = "LightBlue"; |
7. Add the following code to the load handler to create a network request that loads the Json file from the server:
JavaScript
Copy Code
|
---|
// pretend we are calling a web service var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function () { }; xhttp.open("GET", "Tutorial2.txt", true); xhttp.send(); |
8. Implement the onreadystatechange handler to parse the json file:
JavaScript
Copy Code
|
---|
if (this.readyState == 4 && this.status == 200) { var graph = JSON.parse(xhttp.responseText); buildDiagram(graph); } |
9. Add the buildDiagram function that creates the tree root node, and call the addActivities function, which builds the tree recursively:
JavaScript
Copy Code
|
---|
function buildDiagram(graph) { // load activity data and create var project = graph.project; var diagramNode = diagram.factory.createShapeNode(bounds); diagramNode.text = project.name; diagramNode.brush = brush; if (project.activities) addActivities(diagramNode, project); } |
10. Define addActivities as follows. It takes as parameters the parent DiagramNode and parent JSON element, iterates the child JSON elements to build the next level of the hierarchy, and creates corresponding diagram items by calling createShapeNode and createDiagramLink.
JavaScript
Copy Code
|
---|
function addActivities(parentNode, parent) { for (var activity of parent.activities) { var diagramNode = diagram.factory.createShapeNode(bounds); diagramNode.text = activity.name; diagramNode.brush = brush; diagram.factory.createDiagramLink(parentNode, diagramNode);
if (activity.activities) addActivities(diagramNode, activity); } } |
11. Use BorderedTreeLayout to arrange the diagram. Add the following code to the end of the buildDiagram function.
JavaScript
Copy Code
|
---|
var layout = new BorderedTreeLayout(); layout.direction = MindFusion.Graphs.LayoutDirection.LeftToRight; layout.linkType = MindFusion.Graphs.TreeLayoutLinkType.Cascading; layout.nodeDistance = 1; layout.levelDistance = -8; // let horizontal positions overlap diagram.arrange(layout); diagram.resizeToFitItems(30); |
12. Publish MindFusion.*.js and tutorial files using web server of your choice. Now you should see this if you open Tutorial2.html in a web browser:
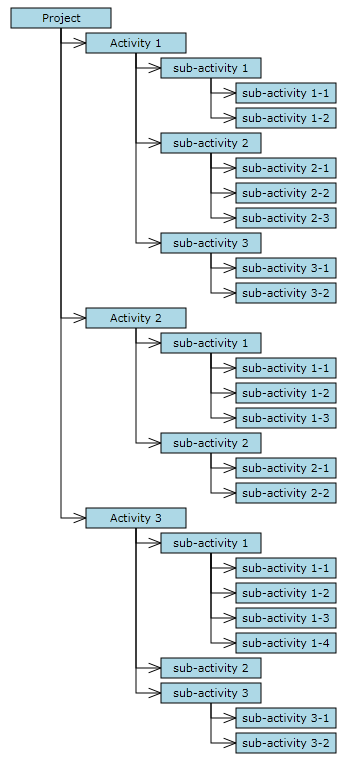