ListView
The ListView control displays a collection of items, which can be arranged vertically or horizontally. The image below shows a vertical list whose items represent people participating in a chat:
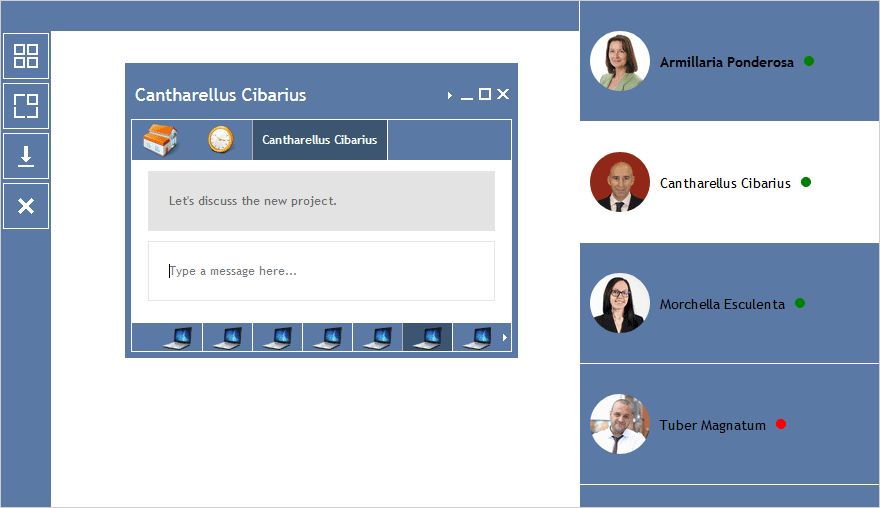
1. Accessing, adding and removing list items
Use view' items property to get a reference to the control's collection of ListItems. Use add and remove methods of the items collection to add and remove list items.
The following code creates a ListView, adds two items and render-s the list:
JavaScript
Copy Code
|
---|
var ui = MindFusion.UI; var listView; var theme = "business"; listView = new ui.ListView(document.getElementById("listView")); listView.width = listView.height = ui.Unit.percentage(100); listView.theme = theme; listView.itemSize = ui.Unit.pixel(100); var item = new ui.ListItem(); item.title = "Tuber Magnatum"; item.data = 1; item.template = generateTemplate("h4.png", item.title, false); listView.items.add(item); item = new ui.ListItem(); item.title = "Morchella Esculenta"; item.template = generateTemplate("h3.png", item.title, true); listView.items.add(item); listView.render(); |
Like other
Control classes from
MindFusion.Common.UI, a
ListView need to be associated with an
HTMLElement. In most cases this is a
div:
HTML
Copy Code
|
---|
<div style="position: absolute; top: 0px; right: 0px; bottom: 0px; width:300px;"> <div id="listView"> </div> </div> <script src="Scripts/MindFusion.UI.js" type="text/javascript"></script> </body> </html> |
Items can also be created by loading a data object or a JSON string, containing the items data, by using the fromObject and fromJson methods respectively.
2. Selecting items
Items can be selected and deselected programmatically by using the
selectItem and
deselectItem methods.
JavaScript
Copy Code
|
---|
//select the second item in the list if(listView.items.count() > 2) listView.selectItem(listView.items.items()[1]); |
3. Customization
The orientation property specifies how list items are arranged - vertically (the default), or horizontally.
JavaScript
Copy Code
|
---|
listView.orientation = MindFusion.UI.Orientation.Horizontal; |
Depending on the orientation the itemSize property can be used to control either the height (for vertical orientation), or the width of the list items. By default, the control allows selection of multiple items when the Ctrl key is pressed, and this behavior can be turned off through the allowMultipleSelection property.
JavaScript
Copy Code
|
---|
listView.allowMultipleSelection = false; |
The appearance of the control can be modified by setting its
theme and
cssClass properties.
4. Drag and drop
Drag and drop capabilities are enabled by default, and can be disabled on a control level by setting the allowDrag property to false.
JavaScript
Copy Code
|
---|
//disables drag and drop for the whole list listView.allowDrag = false; |
To disable drag operations only for a specific ListItem, set its interactive property to false.
JavaScript
Copy Code
|
---|
//disable drag for this list item only listItem.interactive = false; |
5. Events
Important events for the
ListView include:
- selectionChanging - a validation event, raised when the selection collection is changing.
- selectionChanged - a notification event, raised when the selected collection is changed.
- itemClick - a notification event, raised when a list item is clicked.
The following code attaches an event handler for the itemDoubleClick event:
JavaScript
Copy Code
|
---|
listView.itemDoubleClick.addEventListener(handleItemDoubleClick);
function handleItemDoubleClick(sender, args) { if (args.item.data) { var tip = new ui.Tooltip(args.item.element); var t = document.createElement("div"); var img = document.createElement("img"); img.width = img.height = "40"; img.src = "icon_fish.png"; img.style.verticalAlign = "middle"; t.appendChild(img); var span = document.createElement("span"); span.innerText = "This user is not currently online"; t.appendChild(span); tip.template = t.outerHTML; tip.theme = "peach"; tip.position= ui.TooltipPosition.Center; tip.trigger = ui.TooltipTrigger.None; tip.render(); tip.show(); args.item.parent.itemMouseLeave.addEventListener(function(){tip.dispose();}); return; } |
6. Serialization
The items data can be serialized to a JSON string via the toJson method and deserialized from a JSON string via the fromJson method.
ListItem
The
ListItem class represents an item in a list control. A list item displays the text, specified by its
title property, and, optionally, an icon, specified by its
imageSrc property, or its content can be set to a custom HTML string via its
template property.
JavaScript
Copy Code
|
---|
var item = new ui.ListItem(); item.title = "Armillaria Ponderosa"; item.visible = false; listView.items.add(item); |